Filters
Filters are implemented from a base mixin class, FiltersMixin
, some examples of the currently implemented
filters are the Bessel filters, BesselFiltersMixin
, Butterworth filters, ButterworthFiltersMixin
and
elliptic filters, EllipticFiltersMixin
.
- class FiltersMixin
Base class for filter mixins for
Signal
derived classes.- __init__(*args, **kwargs)
Class constructor.
- filters_plot(path, signal, *args, begin=None, end=None, munits=0, **kwargs)
Performs a plot of the original signal and the provided filtered signal.
- Parameters:
path (
str
) – The path where to store the plot, seePlotter.plot()
.signal (
Self
) – The filtered signal.args – Additional arguments to pass to the plotting function, see
Plotter.plot()
.begin (
Optional
[float
]) – The begin value of the horizontal axis where the plot starts, seePlotter.plot()
end (
Optional
[float
]) – The end value of the horizontal axis where the plot end, seePlotter.plot()
.munits (
float
) – Margin units for the plot, seePlotter.plot()
kwargs – Additional keyword arguments to pass to the plotting function, see
Plotter.plot()
.
- Return type:
Self
- Returns:
Instance of the class.
- exception FiltersError
Filters exception class.
Bessel Filters
The BesselFiltersMixin
implements a mixin that can be added to Signal
derived classes to
add Bessel filters functionality, as shown in the code snippet below:
import signal_edges.signal as ses
class ExampleSignal(ses.filters.BesselFiltersMixin, ses.Signal):
pass
An example of its usage using VoltageSignal
is described below:
import numpy as np
import signal_edges.signal as ses
# Create timestamps for the signal, and calculate the sampling frequency.
signal_timestamps = np.linspace(start=0, stop=160, num=160, endpoint=False)
sampling_frequency = 1 / (signal_timestamps[1] - signal_timestamps[0])
# Create voltages for the signal, and add some noise to them.
signal_voltages = np.asarray([0, 0, 0, 0, 5, 5, 5, 5, 5, 5] * (160 // 10)) + \
np.random.normal(0, 0.1, 160)
# Create signal.
signal = ses.VoltageSignal(signal_timestamps, signal_voltages, "s", "V")
# Create the filtered signal.
filtered_signal = signal.filters_bessel(sampling_frequency, 2, lp_cutoff=sampling_frequency / 2.5)
# Plot original signal and filtered signal.
signal.filters_plot("signal.png", filtered_signal)
This code snippet generates the following plot:
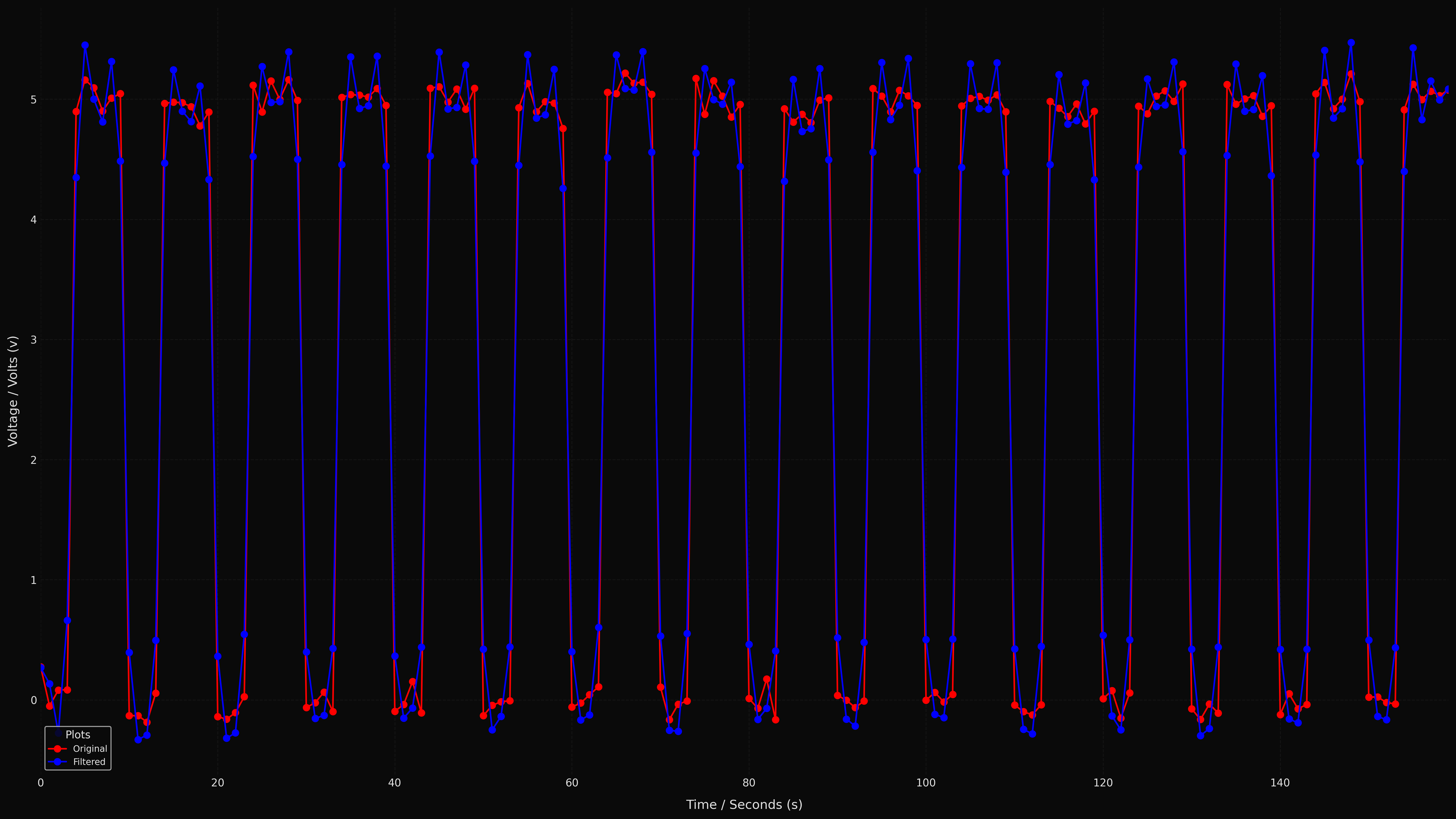
The generated signal and filtered signal in the code snippet.
- class BesselFiltersMixin
Bases:
FiltersMixin
Mixin derived from
FiltersMixin
forSignal
that implements Bessel filters.- filters_bessel(sampling_frequency, order, lp_cutoff=None, hp_cutoff=None, bp_cutoff=None, bs_cutoff=None)
Runs a Bessel filter on the signal, refer to
scipy.signal.bessel()
for more information.One of
lp_cutoff
,hp_cutoff
,bp_cutoff
orbs_cutoff
must be specified. If none or more than one are specified, an error is raised as it won’t be possible to determine the type of filter to apply.- Parameters:
sampling_frequency (
float
) – Sampling frequency in Hertz.order (
int
) – Order of the filter.lp_cutoff (
Optional
[float
]) – Use a lowpass filter with this cutoff frequency in Hertz, defaults toNone
.hp_cutoff (
Optional
[float
]) – Use a highpass filter with this cutoff frequency in Hertz, defaults toNone
.bp_cutoff (
Optional
[tuple
[float
,float
]]) – Use a bandpass filter with this pair of cutoff frequencies in Hertz, defaults toNone
.bs_cutoff (
Optional
[tuple
[float
,float
]]) – Use a bandstop filter with this pair of cutoff frequencies in Hertz, defaults toNone
.
- Raises:
FiltersError – Could not determine the type of filter to use.
FiltersError – Could not create underlying Second Order Sections for filter.
- Return type:
Self
- Returns:
A new instance of the class with the filtered signal.
Butterworth Filters
The ButterworthFiltersMixin
implements a mixin that can be added to Signal
derived classes to
add Butterworth filters functionality, as shown in the code snippet below:
import signal_edges.signal as ses
class ExampleSignal(ses.filters.ButterworthFiltersMixin, ses.Signal):
pass
An example of its usage using VoltageSignal
is described below:
import numpy as np
import signal_edges.signal as ses
# Create timestamps for the signal, and calculate the sampling frequency.
signal_timestamps = np.linspace(start=0, stop=160, num=160, endpoint=False)
sampling_frequency = 1 / (signal_timestamps[1] - signal_timestamps[0])
# Create voltages for the signal, and add some noise to them.
signal_voltages = np.asarray([0, 0, 0, 0, 5, 5, 5, 5, 5, 5] * (160 // 10)) + \
np.random.normal(0, 0.1, 160)
# Create signal.
signal = ses.VoltageSignal(signal_timestamps, signal_voltages, "s", "V")
# Create the filtered signal.
filtered_signal = signal.filters_butterworth(sampling_frequency, 2, lp_cutoff=sampling_frequency / 2.5)
# Plot original signal and filtered signal.
signal.filters_plot("signal.png", filtered_signal)
This code snippet generates the following plot:
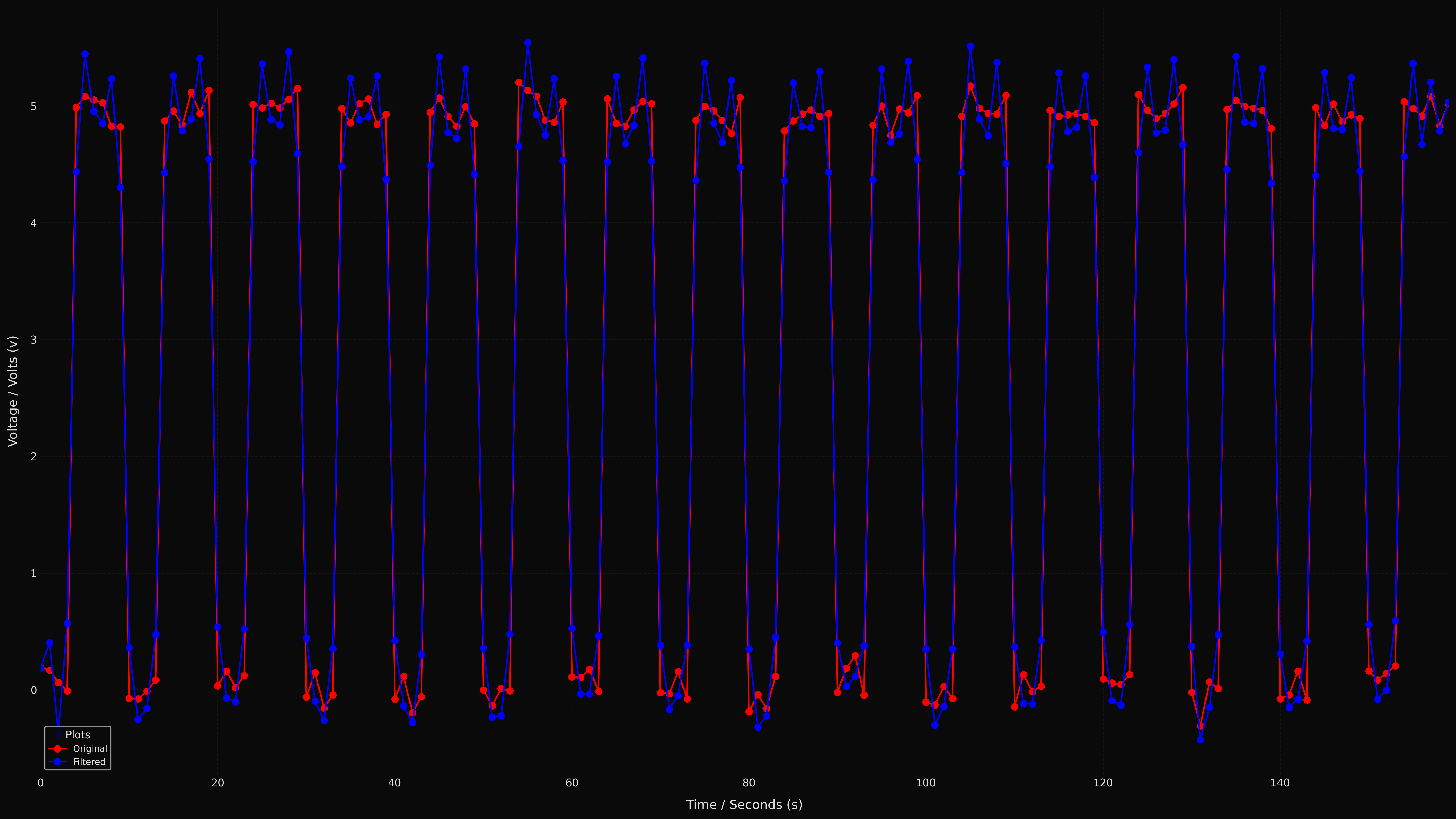
The generated signal and filtered signal in the code snippet.
- class ButterworthFiltersMixin
Bases:
FiltersMixin
Mixin derived from
FiltersMixin
forSignal
that implements Butterworth filters.- filters_butterworth(sampling_frequency, order, lp_cutoff=None, hp_cutoff=None, bp_cutoff=None, bs_cutoff=None)
Runs a Butterworth filter on the signal, refer to
scipy.signal.butter()
for more information.One of
lp_cutoff
,hp_cutoff
,bp_cutoff
orbs_cutoff
must be specified. If none or more than one are specified, an error is raised as it won’t be possible to determine the type of filter to apply.- Parameters:
sampling_frequency (
float
) – Sampling frequency in Hertz.order (
int
) – Order of the filter.lp_cutoff (
Optional
[float
]) – Use a lowpass filter with this cutoff frequency in Hertz, defaults toNone
.hp_cutoff (
Optional
[float
]) – Use a highpass filter with this cutoff frequency in Hertz, defaults toNone
.bp_cutoff (
Optional
[tuple
[float
,float
]]) – Use a bandpass filter with this pair of cutoff frequencies in Hertz, defaults toNone
.bs_cutoff (
Optional
[tuple
[float
,float
]]) – Use a bandstop filter with this pair of cutoff frequencies in Hertz, defaults toNone
.
- Raises:
FiltersError – Could not determine the type of filter to use.
FiltersError – Could not create underlying Second Order Sections for filter.
- Return type:
Self
- Returns:
A new instance of the class with the filtered signal.
Elliptic Filters
The EllipticFiltersMixin
implements a mixin that can be added to Signal
derived classes to
add elliptic filters functionality, as shown in the code snippet below:
import signal_edges.signal as ses
class ExampleSignal(ses.filters.EllipticFiltersMixin, ses.Signal):
pass
An example of its usage using VoltageSignal
is described below:
import numpy as np
import signal_edges.signal as ses
# Create timestamps for the signal, and calculate the sampling frequency.
signal_timestamps = np.linspace(start=0, stop=160, num=160, endpoint=False)
sampling_frequency = 1 / (signal_timestamps[1] - signal_timestamps[0])
# Create voltages for the signal, and add some noise to them.
signal_voltages = np.asarray([0, 0, 0, 0, 5, 5, 5, 5, 5, 5] * (160 // 10)) + \
np.random.normal(0, 0.1, 160)
# Create signal.
signal = ses.VoltageSignal(signal_timestamps, signal_voltages, "s", "V")
# Create the filtered signal.
filtered_signal = signal.filters_elliptic(sampling_frequency, 2, lp_cutoff=sampling_frequency / 2.5)
# Plot original signal and filtered signal.
signal.filters_plot("signal.png", filtered_signal)
This code snippet generates the following plot:
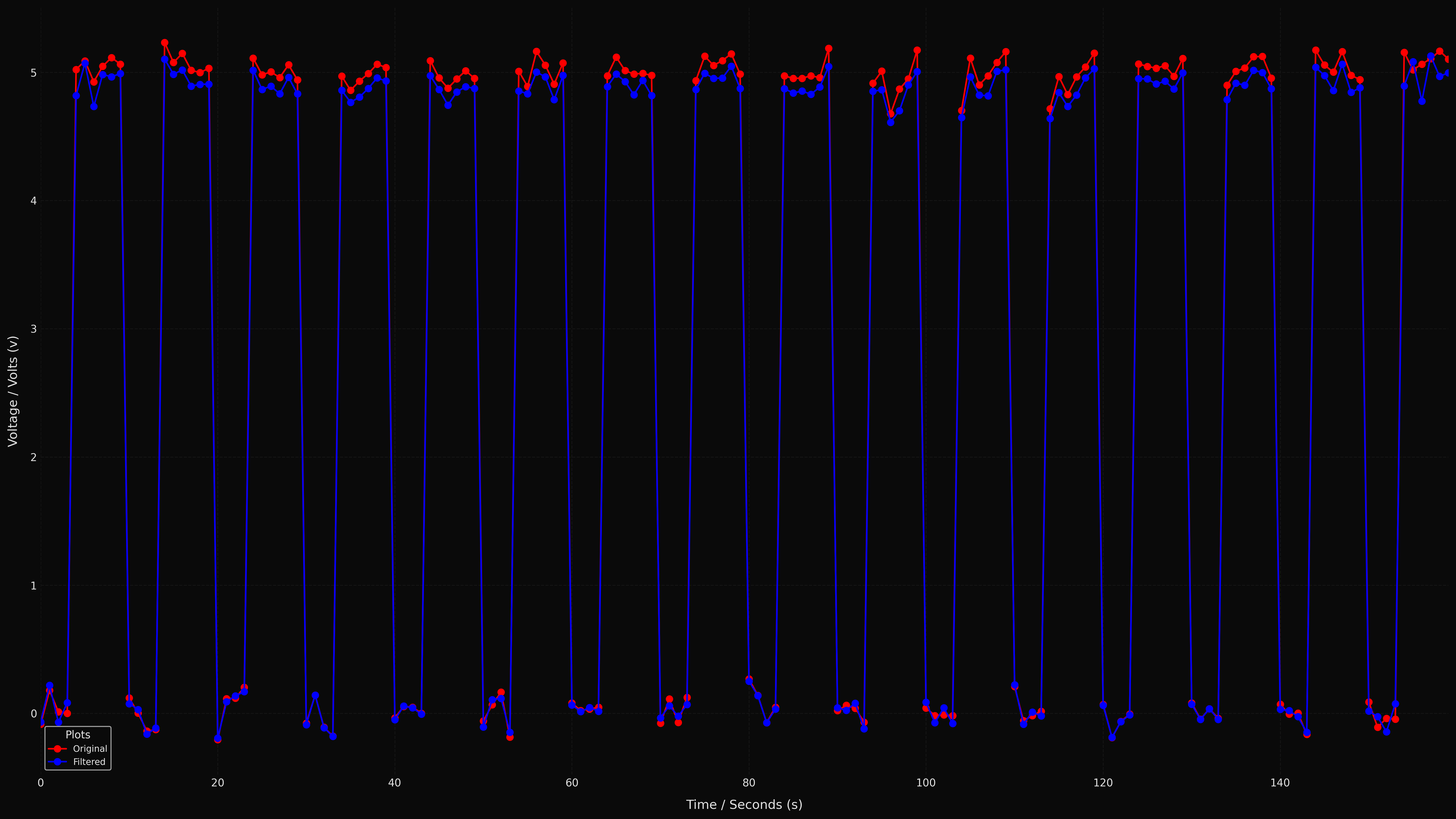
The generated signal and filtered signal in the code snippet.
- class EllipticFiltersMixin
Bases:
FiltersMixin
Mixin derived from
FiltersMixin
forSignal
that implements elliptic filters.- filters_elliptic(sampling_frequency, order, lp_cutoff=None, hp_cutoff=None, bp_cutoff=None, bs_cutoff=None, pb_ripple=0.1, sb_ripple=100.0)
Runs an elliptic filter on the signal, refer to
scipy.signal.ellip()
for more information.One of
lp_cutoff
,hp_cutoff
,bp_cutoff
orbs_cutoff
must be specified. If none or more than one are specified, an error is raised as it won’t be possible to determine the type of filter to apply.- Parameters:
sampling_frequency (
float
) – Sampling frequency in Hertz.order (
int
) – Order of the filter.lp_cutoff (
Optional
[float
]) – Use a lowpass filter with this cutoff frequency in Hertz, defaults toNone
.hp_cutoff (
Optional
[float
]) – Use a highpass filter with this cutoff frequency in Hertz, defaults toNone
.bp_cutoff (
Optional
[tuple
[float
,float
]]) – Use a bandpass filter with this pair of cutoff frequencies in Hertz, defaults toNone
.bs_cutoff (
Optional
[tuple
[float
,float
]]) – Use a bandstop filter with this pair of cutoff frequencies in Hertz, defaults toNone
.pb_ripple (
float
) – Pass band ripple, defaults to0.1
.sb_ripple (
float
) – Stop band ripple, defaults to100.0
.
- Raises:
FiltersError – Could not determine the type of filter to use.
FiltersError – Could not create underlying Second Order Sections for filter.
- Return type:
Self
- Returns:
A new instance of the class with the filtered signal.