Signal Generator
The signal generator, SignalGenerator
, can be used to generate different kinds arrays suitable to create
signals for testing. A code snippet of its use along with VoltageSignal
is shown below:
import signal_edges.signal as ses
# Create generator with initial values.
generator = ses.generator.SignalGenerator(0, 0.001, 100, 0, 100)
# Build signal with multiple pulses.
for _ in range(0, 4):
generator.add_flat(10)
generator.add_edge("falling", 0.0, 10)
generator.add_flat(10)
generator.add_edge("rising", 100.0, 10)
# Generate signal with some noise.
(signal_x, signal_y) = generator.generate(noise=(0, 5))
signal = ses.VoltageSignal(signal_x, signal_y, "s", "V")
# Plot signal.
signal.signal_plot("signal.png")
This code snippet generates the following plot:
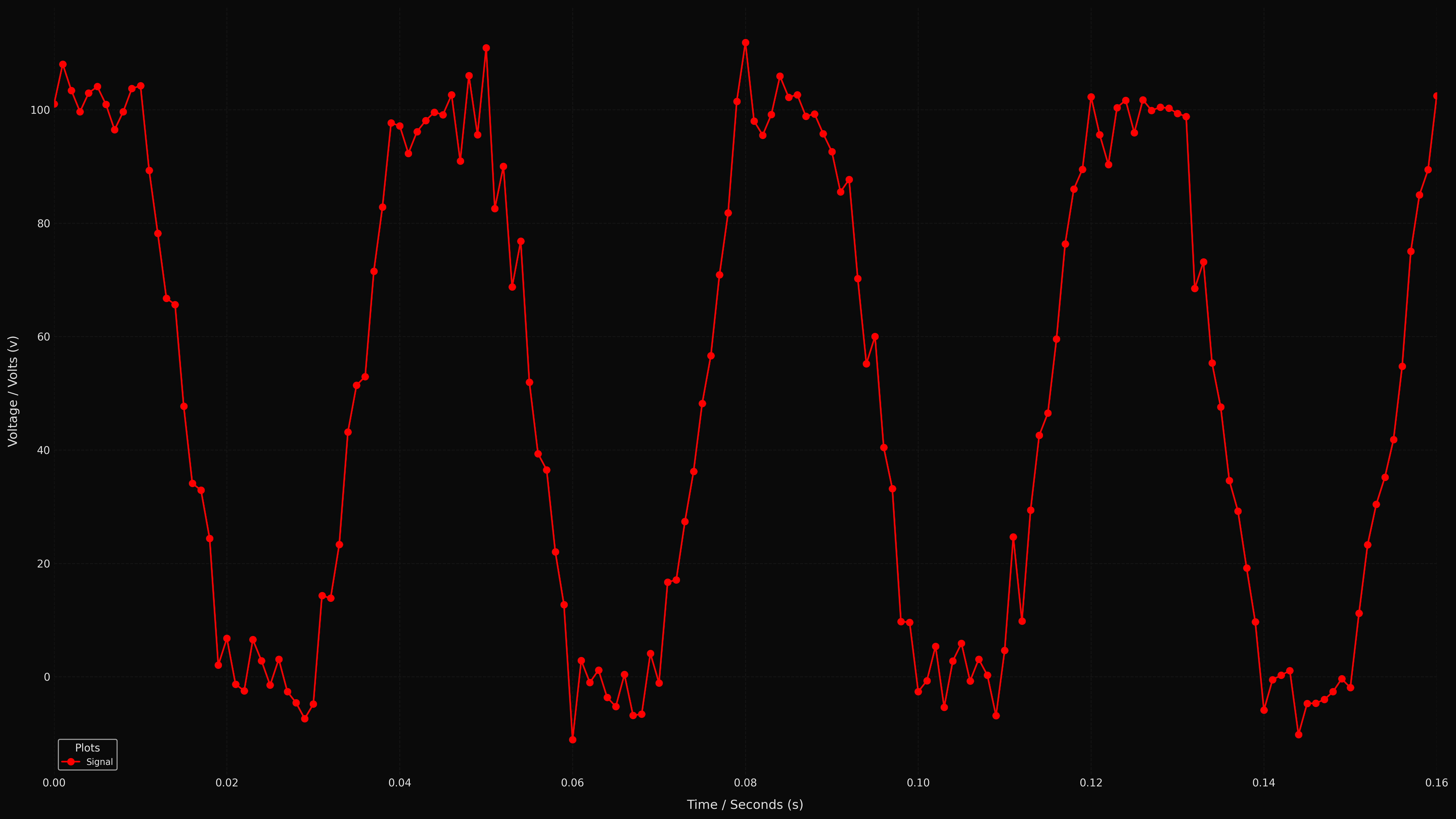
The generated signal.
- class SignalGenerator
Generates arrays for the horizontal axis and vertical axis of
Signal
derived classes.- __init__(hinit, hstep, vhigh, vlow, vinit)
Class constructor.
- Parameters:
hinit (
float
) – Initial value on the horizontal axis.hstep (
float
) – Step between values of the horizontal axis, created automatically as values on the vertical axis are added.vhigh (
float
) – The highest value on the vertical axis, used to calculate the full range of the signal.vlow (
float
) – The lowest value on the vertical axis, used to calculate the full range of the signal.vinit (
float
) – The initial value of the vertical axis, as a percentage of the full range,vhigh
-vlow
.
- Raises:
SignalError – At least one of the parameters given is invalid.
- add_flat(values)
Adds a flat section, using the last value on the vertical axis as reference.
- Parameters:
values (
int
) – Number of values to add to both horizontal and vertical axes.- Raises:
SignalError – The number of values must be larger than zero.
- Return type:
- Returns:
Instance of the class.
- add_edge(edge_type, vtarget, values)
Adds a rising or falling edge.
- Parameters:
- Raises:
SignalError – The number of values must be larger than zero.
SignalError – The type of edge is not valid.
SignalError – The target value must be higher than the current value for a rising edge.
SignalError – The target value must be lower than the current value for a falling edge.
- Return type:
- Returns:
Instance of the class.
- repeat(count=1)
Repeats the current pattern the specified number of times.
- Parameters:
count (
int
) – The number of times to repeat the signal.- Raises:
SignalError – The number of times to repeat the signal not one or higher.
- Return type:
- Returns:
Instance of the class.
- generate(noise=None, hdecs=None, vdecs=None)
Obtains the values for the generated signal, optionally adding Gaussian noise.
- Parameters:
noise (
Optional
[tuple
[float
,float
]]) – Gaussian noise to add, mean and stddev respectively, defaults to no noise.hdecs (
Optional
[int
]) – Number of decimals to round the horizontal axis values to, defaults to no rounding.vdecs (
Optional
[int
]) – Number of decimals to round the vertical axis values to, defaults to no rounding.
- Return type:
tuple
[ndarray
[Any
,dtype
[float64
]],ndarray
[Any
,dtype
[float64
]]]- Returns:
The values of the horizontal axis and the values of the vertical axis.